Coding my dream
Java EE, Spring Framework, SIP ......
Sunday, April 7, 2013
Brief Introduction to Java Classloader
Tuesday, March 26, 2013
Java Concurrency: Fair Lock
java.util.concurrent.locks.ReentrantLock has a constructor with a boolean parameter. With this constructor, you can have fair lock mechanism. ReentrantLock has a queue to contain threads which want to acquire this lock. When a thread releases this lock, then the header will acquire this lock if the queue is not empty.
Tuesday, March 19, 2013
Use jOOQ to mock JDBC
Tuesday, March 12, 2013
SIP Servlet 2.0 POJOs
The link of the draft of SIP Servlet 2.0 POJOs: http://java.net/projects/sipservlet-spec/lists/jsr359-experts/archive/2013-02/message/41
Thursday, March 7, 2013
MMIM MSRP EOF Exception
Template Method pattern in Java - Two Ways
Saturday, March 2, 2013
Java Concurrency: Thread State
In Java, a thread has 5 kinds of states: NEW, RUNNABLE, BLOCKED, WAITING, TIMED_WAITING, TERMINATED. When you execute Thread.sleep(long), the current thread status will transform from RUNNABLE to TIMED_WAITING, and the current thread will give up CPU resources to another threads. But the current thread will not give up the monitor.
When execute Object.wait(), the current thread will become to WAITING state ( Object timed wait will make thread to TIMED_WAITING state ). But different with Thread.sleep(), Object.wait() will make thread to give up the monitor.
When your code use synchronized keyword try to acquire a monitor but blocked, the current thread will be at BLOCKED state. At that moment, the thread cannot respond to the interruption. But at WAITING and TIMED_WAITING state, a thread can have chance to respond to the interruption.
Different with synchronized, Lock.tryLock(long time, TimeUnit unit) can respond to the interruption. So use Lock in Java 5 concurrent is a way to avoid dead lock.
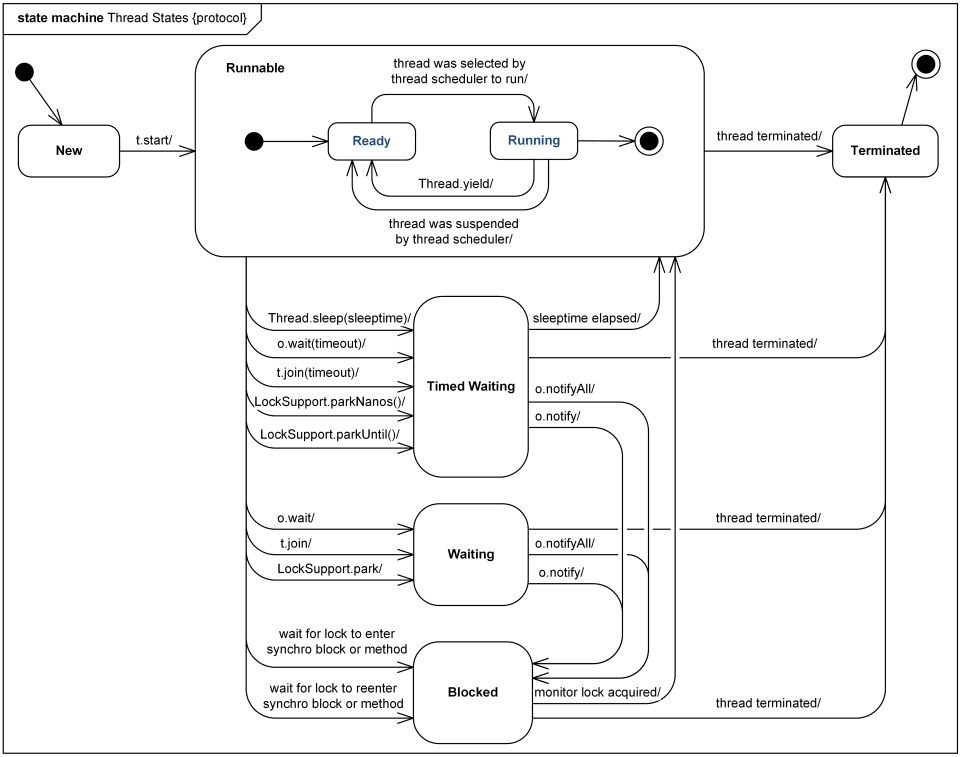